Home Up
| |
BASICS:
Or should I say Visual Basics?
From the time electronic computers were invented
people have had to write program code...to make the hardware do what is
required.
Some things to remember about hardware:
 | Hardware is limited physically |
 | Understanding hardware helps us to design
programs in a better way |
 | Hardware does not understand natural
languages only 0's and 1's |
 | Hardware uses the Binary system or variations
of it to execute instructions, store data and the like. |
 | The Binary System:
Before we even start to consider what a
program is we need to understand how data is stored and manipulated in a
computer:
 | Data is stored in various parts of the
computer hardware |
 | These hardware parts have physical limits
imposed on them by various design constraints
 | this imposes a limit on the size of a
word or number one can represent and store within these hardware parts |
|
 | The size of the data (word or number or
picture) is measured in Bits or Bytes (1 Byte = 8 Bits)
 | 1 Byte is the amount of data required to
represent 1 character in a computer |
 | We also have more types of unit
definitions: |
|
Name |
Exact
Measure |
Approximate
Measure |
KiloByte or KB |
= 1024 Bytes |
1000 Bytes |
MegaByte or MB |
= 1024*1024 Bytes |
1,000,000 Bytes |
GigaByte or GB |
= 1024*1024*1024 Bytes |
1,000,000,000 Bytes |
TeraByte or TB |
= 10244 Bytes |
1,000,000,000,000 Bytes |
 | A bit represents one of two binary states
we usually represent these as 0 or 1
 | it is the smallest unit of storage
capacity used |
 | in reality a bit may be a positive or
negative voltage, a electrostatic charge, a magnetised element,... |
|
 | Bit size to represent data has increased as
technology has improved.
 | Today 32 and 64 bit computing is common
in PCs, super computers use 1024 bits and more to represent individual
pieces of data
 | The more bits you can move around per
CPU operation the faster your PC can perform calculations |
|
|
The use of Bits raises the question of what
decimal number does the binary number stored in a computer 1110011011110110
represent in our number system (decimal)?
Clearly we need to be able to covert a number
defined in a particular number base to decimal number base and visa-versa.
Typical number bases used in computing are as follows:
 | binary - number base 2 |
 | octal - number base 8 |
 | hex - number base 16 |
A table of numbers and their representation in
different number bases:
Decimal |
Hex |
Octal |
Binary |
0 |
0 |
0 |
0 |
1 |
1 |
1 |
1 |
2 |
2 |
2 |
10 |
3 |
3 |
3 |
11 |
4 |
4 |
4 |
100 |
5 |
5 |
5 |
101 |
6 |
6 |
6 |
110 |
7 |
7 |
7 |
111 |
8 |
8 |
10 |
1000 |
9 |
9 |
11 |
1001 |
10 |
A |
12 |
1010 |
11 |
B |
13 |
1011 |
12 |
C |
14 |
1100 |
13 |
D |
15 |
1101 |
14 |
E |
16 |
1110 |
15 |
F |
17 |
1111 |
Examples of number conversion:
 | Convert a number AB12n in a base
n to decimal = A*n3 + B*n2 +1*n1 +2*n0 |
 | Convert 123410 to an equivalent
number in base 16
 | 1234 / 16 = 77 rem 2 |
 | 77 / 16 = 4 rem 13 |
 | 4 / 16 = 0 rem 4 |
|
Therefore 123410 = 4D216
PROGRAMMING:
 | We assume here that we are using sequential
processing rather than parallel processing to solve our problems. |
 | An application (program) is a set of
ordered instructions to the CPU telling it what to do and is a solution to a
particular problem or set of problems |
 | Programs that are "running" are usually
stored in RAM |
 | When an instruction is sent to the control
unit (see CPU diagram) it is “Fetched”, Decoded to CPU language and Executed
this is called the F-D-E cycle |
 | Instructions are executed one at a time and
in some specific sequence |
TYPICAL STRUCTURE OF A CPU
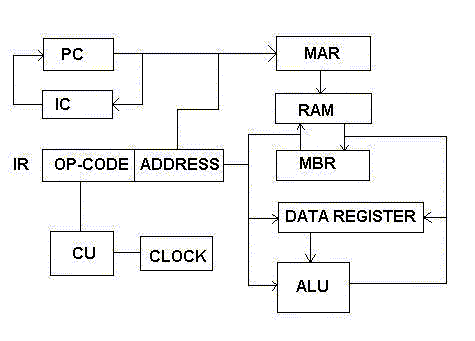
 | More than one program can be stored in RAM
and the CPU has to switch between the instructions of each program doing the
F-D-E cycle. This switching process is called “Multitasking”. |
 | However because the CPU is so fast it seems
to us that instructions (programs) are executed in parallel BUT this is NOT
parallel processing. |
 | Parallel processing is when there is more
than one CPU performing different tasks of the same program(s) at the same
time. |
Instructions (code) can be written in
different languages
 | Machine Code most basic code combination of
1 & 0 s
 | but difficult for humans to understand |
|
 | Assembler Code, different types depends on
brand of CPU (i.e. Motorola, Intel)
 | easier than machine code but still far
from the usual way people express problems |
|
 | High Level Languages Visual Basic, C, C++,
Pascal, Java,…
 | easier than the previous types closer to
how people express problems |
|
Programs are not just written the following
steps need to take place, This process is called the Program Development Life
Cycle (PDLC)
 | Analysis of the “problem” |
 | Design of the program using some method
(methodology Top Down, Bottom Up, OOD,…) |
 | Coding of the program |
 | Testing of the program |
 | Documentation |
 | Maintenance of Program |
Structured programming or structured design
 | Is a methodology to help translate a
problem into a program |
 | We can use flowcharts, to help us get the
solution in a graphical way |
 | We can use pseudo-code, a set of
instructions written in your own spoken language |
Example of a Flowchart:
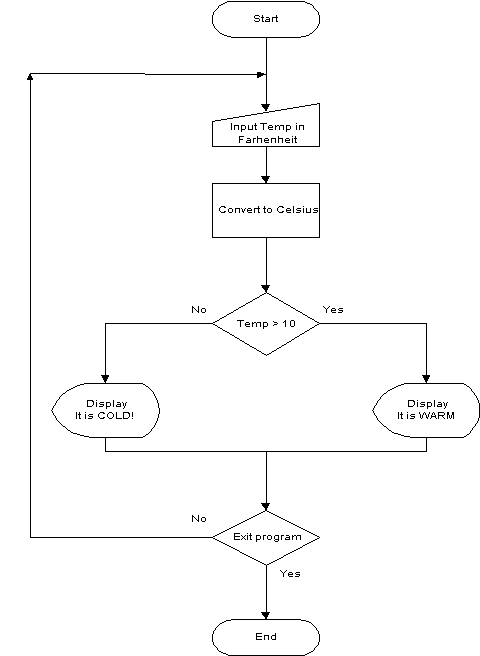
The set of steps of a solution to a problem
is called an algorithm:
There exists the idea of a General
Algorithm which has 5 main steps
- Start (define variables, constants,…)
- Input (Data)
- Process (Data)
- Output (Data, Information = Results)
- End (= exit)
We can break down a problem into these 5 steps
at different levels of abstraction
Some code structures we use again and
again in many different
programming languages the most common are as follows:
The Select Case is similar to the IF-ELSEIF-...-ELSE-END
IF
 | Select Case test-expression |
 | Case expression1 |
 | 'do these code
statements |
 | Case expression2 |
 | 'do these code
statements |
 | Case Else |
 | 'do these code
statements |
 | End Select |
Do - Until control structures are
used when we DON'T KNOW exactly the numbers of times we want to execute the
code inside the loop
 | Do Until User_Input = "x" Or "X" |
 | Print Enter a number to add to
previous TO Exit type x or X |
 | j = Val(Txt_Num1.Text) |
 | Sum = Sum + j |
 | Loop |
 | Array control structures give us the
power to store similar types of data together and be able to use the data
quickly.
 | Lbl_Name(0).Caption = "John" |
 | Lbl_Name(9).Caption = "Mary" |
|
The thing to remember is
that programs are designed to work NOT just written with the hope that they will
work!!
 | EXAMPLE of the design steps of a simple
program
|
You are to design
a program that performs the following:
The user enters (types)
the radius of a circle and the program calculates the area of the circle and
displays the result on screen.
Before you start your
design you can make a sketch on paper of what you think is required:
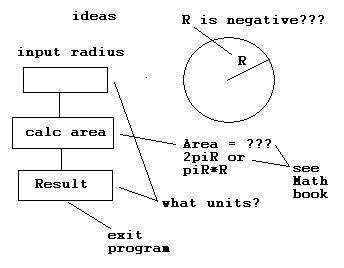
In your design you can
use either Pseudo-Code or a Flow Chart remember. Note that the General
Algorithm has the following steps:
-
Start
-
Input
-
Processing
-
Output
-
End
A SOLUTION to the
Calculate Area program USING PSEUDO CODE:
 |
LEVEL 1 PSEUDO CODE:
 |
1. Start |
 |
2. Input |
 |
2.1 Display message to user to enter the
radius and what units are used |
 |
3. Process |
 |
3.1 Calculate area of circle |
 |
|
 |
|
 |
5. End |
|
 |
LEVEL 2 PSEUDO CODE:
After some more thought and questions!!!
 |
1. Start
 |
define PI as a
constant |
|
 |
2. Input
 |
2.1 Display message to user to enter the
radius |
 |
2.2 Display units of Radius |
|
 |
3. Process
 |
3.1 Calculate area of circle using Area =
PI*Radius2 |
|
 |
|
 |
5. End |
|
 |
LEVEL 3 PSEUDO CODE:
Is after even more thought and we start to introduce code statements |
 |
1. Start
 |
1.1
Use form, labels, textbox, command button
objects for interface |
|
 |
 |
2.1 Display message to user to enter the
radius |
 |
2.1.1 Use Label1 object to display message
mentioned in 2.1 |
 |
2.2 Display units of radius |
 |
2.2.1 Use Label1 object to display message
mentioned in 2.2 |
 |
2.2.2 Set .Caption in Properties window to
-> Enter the radius in cm |
|
 |
3. Process
 |
3.1 Calculate area of circle using Area =
PI*Radius2
|
 |
3.1.1 Use Text1 object to get value of
Radius |
 |
3.1.1.1 Use .Text property of object
Text1 |
 |
3.1.1.2 Set the .Text property in
Properties window to blank |
 |
3.1.2 Use formula Area = PI *Val
(TxtRadius .Text)^2 inside Command Button named Command1
 |
a better name for Command1 control
is e.g. CmdArea |
|
|
 |
|
 |
5 End |
Example of VB6 Form
and Code
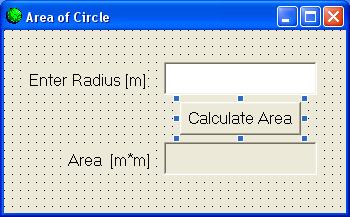
Const PI = 3.141591 'defined in General
Private Sub CmdArea_Click()
LblArea.Caption = PI * Val(TxtRadius.Text) ^ 2
End Sub
So what would be the next
refinement of the design above?
|
|